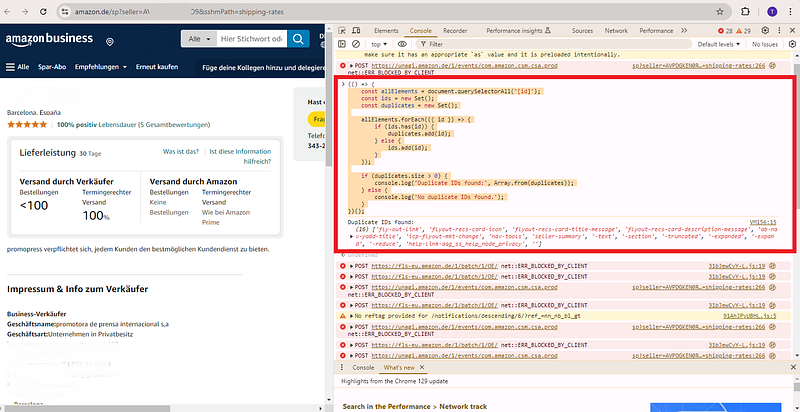
Duplicate IDs in HTML are problematic because the id
attribute must be unique. If you’re working on a complex web project, it’s easy to unintentionally assign the same id
to multiple elements, which can cause unexpected behavior in your scripts or styles.
Luckily, finding duplicate IDs in your DOM can be a breeze using JavaScript in the Chrome Developer Tools. In this quick guide, I’ll show you a simple way to do it.
Step 1: Open Chrome Developer Tools
To get started, right-click on the page and select Inspect or press Ctrl + Shift + I
(Windows) or Cmd + Option + I
(Mac) to open the Developer Tools.
Step 2: Use the Console to Run a Script
In the Developer Tools, switch to the Console tab. Paste the following JavaScript snippet into the console and press Enter:
(() => {
const allElements = document.querySelectorAll('[id]');
const ids = new Set();
const duplicates = new Set();
allElements.forEach(({ id }) => {
if (ids.has(id)) {
duplicates.add(id);
} else {
ids.add(id);
}
});
if (duplicates.size > 0) {
console.log('Duplicate IDs found:', Array.from(duplicates));
} else {
console.log('No duplicate IDs found.');
}
})();
Step 3: Check for Duplicates
After running the script, the console will either display a list of duplicate IDs or a message saying no duplicates were found.
Why This Matters
Having duplicate IDs can lead to CSS and JavaScript issues because the id
attribute is meant to be unique within a page. This script helps ensure your HTML is clean and follows best practices.
Final Thoughts
Finding duplicate IDs is just one step in maintaining clean and functional HTML. Whether you’re debugging a layout or tracking down an odd JavaScript bug, this simple script can save you time.
Happy coding!